1. I have a penchant for killing plants.
2. People in Holland grow things really well indoors.
After reading about how well things can grow indoors, I started thinking that maybe automation was my path to healthy plants. So I decided to build the bare minimum - get a plant, a pump, and a water sensor. When the water sensor says "no water here", use the pump to put water there.
I also decided to run it all through a Raspberry Pi to as an excuse to interact with the RPi GPIO.
Here's how I did it!
Materials:
- Raspberry Pi 3
- Soil Moisture Sensor
- Flexible Water Line
- 5V Relay
- 3-6V Mini Micro Submersible Pump
- TOLI 120pcs Multicolored Dupont Wire
- 5v Power Supply (Any USB Cable+ USB Wall Charger)
Wiring:
Now time for the GPIO.
RPi Wiring:
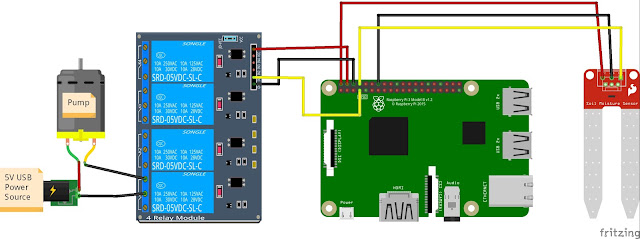
Following this GPIO layout:
Water Sensor - plug the positive lead from the water sensor to pin 2, and the negative lead to pin 6. Plug the signal wire (yellow) to pin 8.
Relay - Plug the positive lead from pin 7 to IN1 on the Relay Board. Also connect Pin 2 to VCC, and Pin 5 to GND on the Relay board.
Pump - Connect your pump to a power source, run the black ground wire between slots B and C of relay module 1 (when the RPi sends a LOW signal of 0v to pin 1, this will close the circuit turning on the pump).
This diagram should capture the correct GPIO so long as you are using Raspberry Pi 3. Not shown is another power source to the RPi.
Hardware Setup:
Once the wiring has been completed, attach the flexible hose to the pump (I used electrical tape), and drop it into a jar of water. Attach the other end of the hose to your plant.
Now plug in all power sources (and make sure your Raspberry Pi is running some version of an operating system, like this one here).
Now plug in all power sources (and make sure your Raspberry Pi is running some version of an operating system, like this one here).
Software
Note: If you get the wiring exactly as described above, my code in the next section will work with no modifications.
There are two parts to this setup. One file controls all the GPIO and circuit logic, and the other runs a local web server.
All files:
water.py
web_plants.py
auto_water.py
main.html
Let's start with the code for controlling the GPIO. This requires the RPi.GPIO python library which can be installed on your Raspberry Pi as follows:
$> python3.4 -m pip install RPi.GPIO
All files:
water.py
web_plants.py
auto_water.py
main.html
GPIO Script
$> python3.4 -m pip install RPi.GPIO
With that installed, you should be able to use the water.py script found here.
You can test this is working correctly by running an interactive python session as follows:
$> python3.4
>>> import water
>>> water.get_status()
>>> water.pump_on()
This should print a statement about whether your sensor is wet or dry (get_status()), and also turn on the pump for 1s. If these work as expected, you're in good shape.
At this point you can also calibrate your water sensor. If your plant status is incorrect, try turning the small screw (potentiometer) on the sensor while it is in moist soil until the 2nd light comes on.
Flask Webserver
The next aspect of this project is to setup the web server. This code can be found here in a file called web_plants.py. This python script runs a web server enabling various actions from the script described above.
You will need to keep web_plants.py in the same directory as water.py described above, as well as auto_water.py. You will also need a sub-directory called "templates" containing the html file here called main.html.
You will need to install flask, and psutil as follows:
$> python3.4 -m pip install flask
$> python3.4 -m pip install psutil
You will need to keep web_plants.py in the same directory as water.py described above, as well as auto_water.py. You will also need a sub-directory called "templates" containing the html file here called main.html.
You will need to install flask, and psutil as follows:
$> python3.4 -m pip install flask
$> python3.4 -m pip install psutil
You will also need to create a sub-directory called templates, and place main.html in the templates directory.
$> sudo python3.4 web_plants.py
Now if you navigate to the ip address of your RPi, you should see a web dashboard something like this:
here's another great tutorial I followed on flask + GPIO
Run Website Automatically
Finally, you probably want the website to auto start when the RPi gets turned on. This can be done using a tool called cronjob, which registers your website as a startup command.
To do so, type:
$> sudo crontab -e
This will bring up a text editor. Add a single line that reads (and make sure to leave one empty line below):
@reboot cd <your path to web_plants>; sudo python3.4 web_plants.py
Now when you reboot your pi, it should auto start the server.
This comment has been removed by the author.
ReplyDeleteCan we use Solenoid valve instead of mini micro submersible pump, Sir?
ReplyDeleteSame question sir.. i would like to use 12v solenoid valve to handle more power. Thanks in advance
ReplyDeleteYes you could use a solenoid valve, but the water would still have to be moved somehow. I have been thinking a gravity fed water line with a solenoid valve would be the best solution for something like a garden. Good luck.
ReplyDeleteSir, solenoid valve needs a 12v source. It is ok to connect it to the 5v relay module?
ReplyDeleteConnecting the 12v source to 5v relay module, instead of 5v source that you use to the pump ...
ReplyDeleteI don't know! Welcome to the joy of DIY :-)
ReplyDeleteIf it's 12V in the circuit the relay closes you should be fine though. If you're powering the relay itself with 12V, probably not good for the longevity of the component.
Sir, for the Soil moisture what is the exact pin you use? The Digital Output or Analog Output?
ReplyDeleteI'm getting a "ImportError: No module named psutil" when im trying to run the web_plants.py even i already installed the module psutil, any advice for this?
ReplyDeleteCheck your folder...
DeleteIf you install it in python then open it in python, if python3.5 open in python 3.5
Could really use clarification on this. Can you provide something more detailed as this is all I cannot get past.
DeleteDanny is saying psutil was probably installed to a different version of python, which could happen if you use "python" to invoke any of these scripts instead of "python3.4".
DeleteTry running all the commands above except with "python3" instead of "python3.4" - this will make it more flexible to your default version of python3.
Hope this helps others... learned I needed to use "sudo python3 -m pip install psutil" to fix it.
DeleteMany thanks Ben!
DeleteHad the same challenge and after spending several hours troubleshooting, I followed your suggested solution using "sudo python3.5 -m pip install --upgrade psutil".
It's working now.
I am testing the auto-water feature but the consecutive_water_count does not remain at 0 when I leave the sensor out on a napkin. I wanted to see how long the pump would keep activating until the consecutive_water_count reached 4 (starting at 0) - knowing fully that it would never reach because I don't have it in water.
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteThanks for all the details. Can this setup be extended via WiFi as iot.what hardware/board can be used.
ReplyDeleteCan you supply power to both the pi and the pump with the 5v? Or will the pi need to be plugged into main somewhere?
ReplyDeletecan you give me report please?
ReplyDeleteHi, How would this work if it was controlled from an Android App?
ReplyDeleteGreat project! I followed it and got it to work as well.... nice! Could you help me understand the soil humidity sensor though please? In the diagram a 3 pin sensor is used, in your vids you a different one with 2 pins... I have ordered such one, but I don't know how to connect and properly calibrate.
ReplyDeleteSame problem here!! Did you ever figure it out?
DeleteSo I think the two wires you're seeing are just extensions of the circuit between the moisture pins... They connect to a tiny pcb with an LED on it where the sensitivity of the sensor is calibrated, and then 3 wires come from that tiny PCB back to the Pi. So its like in the diagram, but with wires running to the prongs of the sensor. Basically the sensor needs power (red and black) and a signal (yellow). Your red and black (VCC/GND) should connect to the input voltage, and yellow(OUT) should go to the RPi GPIO pin 8.
DeleteHi again! It turns out the potentiometer had 4 pin outputs but you only have to wire 3 to the raspbery pi as one is digital output (DO) and one is analog output (AO) as we are using digital hardware, you just wire the ground, VCC (power) and DO (digitaloutput) Thanks for your help though! Currently testing mine on the floor!
Deletevery nice one sir..
ReplyDeletePlzz someone explain those connections of youtube clearly
ReplyDeleteWhen I turn on auto watering. and I put my soil moisture sensor in a dry condition the auto watering said its ON but the pump does not pump the water
ReplyDeleteSo I think I have everything working. The web server runs upon bootup, but nothing seems to automatically trigger the "auto-watering" command. I have to load the main.html page and click the button to get things started. This won't work as a fully-automated solution in the event of a power outage.
ReplyDeleteWhere would I put a call to have the auto-watering default to "ON" whenever the web server starts up on a reboot? What would that call look like?
Oops! I put that as a reply to Danny's comment. Meant to make this a general comment.
ReplyDeleteEverything is working. The web server runs upon bootup. However, it does NOT default to "auto water ON". That must be manually triggered.
I need the program to default to auto-watering ON as there will indeed be a power outage.
Where would I put a call to have the auto-watering default to "ON" whenever the web server starts up on a reboot? What would that call look like?
I've tried putting the call of "water.auto_water()" in "web_plants.py". While that works, then the web server won't run. It seems to be one works or the other, but not both.
Thank you. This is great.
ReplyDelete"Physical" pin 7 is BCM pin 4 so this setup worked for me
GPIO.setmode(GPIO.BCM)
pump = 4 # not 7 because we're setting the mode to BCM
GPIO.setup(pump, GPIO.OUT)
how would you set this up for multiple plants? Thanks! :)
ReplyDeleteI am having issues with auto watering.
ReplyDeleteI have everything working correctly . I couldn’t get cron to work so I added the web_plants.py to the raspy-config file, and it runs fine in every boot.
But..
When I click on auto water. The pump runs 5 times, then stops. Which I think is what it is supposed to do based on the script, and then it never runs again.
I have my sensor out, so in a dry place. I would expect to retrouver the auto watering after a delay.
But it only run 5 times. Then, I can go to the webpage, read the sensor (1) and re enable auto watering. Then it triggers the pump 5 times again. And stops again
What am I missing that is preventing my setup to run correctly ?
Ok, I think I understand a little more. Your 5 count on auto watering is there to prevent the pump from running on empty ?
ReplyDeleteIf so, the 5 pump triggers should be sufficient to moist the area where the sensor is.
It is working fine now that I understand this. I set the consecutive pumps to 10.
Next step is to use a capacitive sensor. Any pointer to do this ?
Marc, nice progress reverse engineering my logic there. Do you have a capacitive sensor in mind?
DeleteWell these are fairly common it seems on Amazon.
ReplyDeleteHttps://www.amazon.com/Gikfun-Capacitive-Corrosion-Resistant-Detection/dp/B07H3P1NRM/ref=sr_1_10?keywords=Capacitive+humidity+sensor+raspberry&qid=1563331508&s=gateway&sr=8-10
Any recommendation ?
Hi, I'm new to all this. Am I supposed to download Raspberry's operating system then run these scripts as executables?
ReplyDeleteHi,
ReplyDeleteI'm quite new to this. Do Raspberry need some extra power suply , not shown in diagram ?
please provide entire code
ReplyDeleteHallo,
ReplyDeleteWhat is this one used for?
TOLI 120pcs Multicolored Dupont Wire
When I run "water once" the pump comes ON and never goes OFF again. How do I stop the pump, please?
ReplyDeleteIf I "turn ON auto watering", the pump comes ON for 5sec, goes OFF for 1sec, then comes ON for 5sec repeatedly. It will then stay ON continuously when sensor detects moisture. Not even "turn OFF auto watering" can stop it. What should I do please.
Every other thing works fine. I just need to fix the above situation.
Help please.